In this tutorial, you will learn to perform temperature sensor data logging to the flash memory of Raspberry Pi Pico W using MicroPython code. In our previous article, we learned how to store data permanently to the flash of Pico W. There, we used JSON format to store data. This article will guide you to save sensor data to a text(.txt) file.
Data such as variables are stored in the RAM (Random-access memory). The information in RAM is lost when the microcontroller is reset or rebooted. So if we want to store data permanently, we have to use a non-volatile form of storage such as an SD card, EEPROM, or flash memory.
Raspberry Pi Pico W has 2MB of onboard flash storage which shall be used to log sensor data.
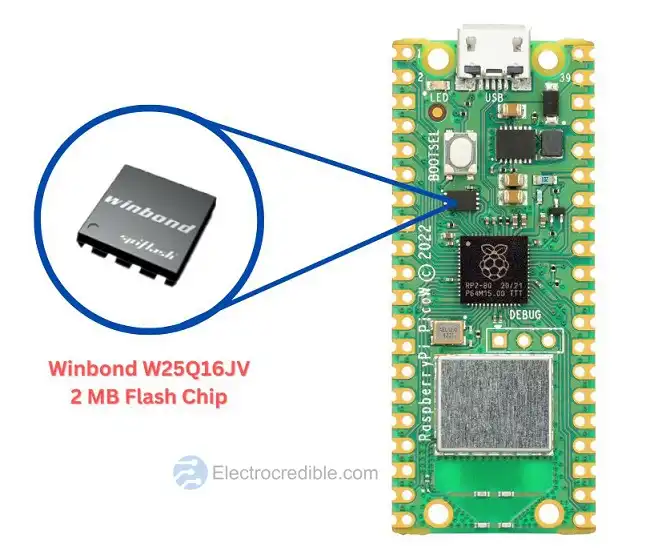
Prerequisites
Your Raspberry Pi Pico needs to be flased with a MicroPython UF2 file to program it in MicroPython code. You can read our getting started guide for Raspberry Pi Pico where we show all steps required to start programming RP2040 in MicroPython.
If you are using macOS, follow our guide to program Raspberry Pi Pico on macOS using Thonny IDE.
How to Store Text Files on Flash Memory of Pico W
First, let us learn how to open/create a text file and store data using MicroPython on Pico W. MicroPython, a subset of Python, allows us to do file operations such as read, write, and append.
To open a file, we can use the inbuilt MicroPython function open(filename, mode)
. The mode
specifies the kind of read and write operations such as:
- w: Opens an existing file to write data. If the file is not present in the directory, then it also creates the file with the filename.
- a: Opens an existing file to append data. Also creates a new file with the filename if the file does not exist.
- r: Open a file to perform a read operation.
Here is an example code to append some text data to a text file.
import os
with open('textdata.txt', 'a') as f:
f.write('This is line 1')
f.write('\n')
f.write('This is line 2')
Code language: Python (python)
If you are using Thonny IDE go to File>New and paste the code above. Run the code and save it to Raspberry Pi Pico as test.py or any other name with a “.py” extension.
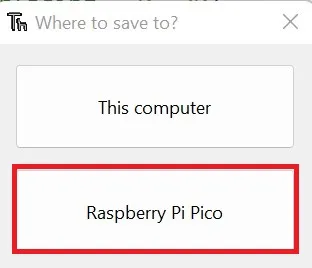
When you run the code, a new file called textdata.txt will be created in the flash memory of Pico W. You can open the file in Thonny IDE and view its text contents. If the files in Pico W are not visible, click on View and mark the option Files.

The file will contain two lines of text as shown in the image below.

Code explanation
We first import the os
module which helps to do file operations. The line with open('textdata.txt', 'a') as f:
opens the file named “textdata.txt” in “append” mode using a with
statement. The a
argument specifies that the file should be opened for appending. The with
statement ensures that the file is properly closed after it is used, even if an exception occurs. The file object is assigned to the variable f
.
We then use the line f.write('This is line 1')
to write the string “This is line 1” to the file f
. The write()
method is called on the file object f
to write content to the file.
f.write('\n')
writes a newline character to the file, which ensures that the next line will be written on a new line. Finally, we write the string “This is line 2” to the file.
Raspberry Pi Pico W Temperature Data Logger
Let us now try to write some simple MicroPython code to log temperature data on the flash memory of Raspberry Pi Pico W. The data will be saved to a new text file.
Taking into consideration the limited size of the flash memory, the code ensures that the data entry occurs only when the file size remains less than a certain limit (20KB in this example). Also, data is logged every two minutes. You can change these parameters in code.
Since this project uses the internal RTC, Raspberry Pi Pico has to be connected to your computer for accurate timestamps. If you would like to make a portable data logger, read our guide on powering Raspberry Pi Pico with batteries and use external RTC modules which are also battery-powered. Read:
- Interface DS3231 With Raspberry Pi Pico-MicroPython/Arduino Code.
- Raspberry Pi Pico RTC DS1302 Tutorial- MicroPython Code.
MicroPython Code For Data Logging
Open Thonny IDE and paste the following code in a new script by going to File>New.
from machine import ADC, RTC
import os
import time
adc = machine.ADC(4)
rtc=RTC()
timeStamp=0
with open('savedata.txt', 'a') as f:
f.write('Temperature Data Logging')
f.write('\n')
def calcTemp():
global temperature_celcius
ADC_voltage = adc.read_u16() * (3.3 / (65535))
temperature_celcius = 27 - (ADC_voltage - 0.706)/0.001721
def logData():
timeTuple=rtc.datetime()
file_size=os.stat('/savedata.txt')
if(file_size[6]<20000):
try:
with open('savedata.txt', 'a') as f:
f.write('Timestamp='+ str(timeTuple[4])+':'+str(timeTuple[5])+',')
f.write('Temperature='+ str(temperature_celcius))
f.write('\n')
except:
print("Error! Could not save")
calcTemp()
logData()
while True:
if (time.ticks_ms()-timeStamp)>120000:
calcTemp()
logData()
timeStamp=time.ticks_ms()
Code language: Python (python)
Save the code to your Raspberry Pi Pico W as main.py. The code will then run automatically when the microcontroller reboots.
You can view the logged temperature data in Thonny IDE by opening the file savedata.txt from the file viewer (If the file viewer is not visible, click on View and mark the option Files).

The image below shows how the logged data will look like.

Note that the Real Time Clock might not show the correct time according to your time zone. You will have to rely on a battery-operated external RTC such as the DS1302 RTC IC. Read- Raspberry Pi Pico RTC DS1302 Tutorial- MicroPython Code.
Data Logging Code Explained
After importing the necessary MicroPython modules in the initial lines, we create an ADC object to read analog values from GPIO 4 of Pico W. This pin is connected to the built-in temperature sensor of Raspberry Pi Pico. (Also read: How to use ADC in RPi Pico W.)
adc = machine.ADC(4)
An RTC object is also created to work with the real-time clock.
rtc=RTC()
A global variable timeStamp
is initialized to zero which will be used to show the time in which temperature readings are taken.
timeStamp=0
The next lines of code open the file “savedata.txt” in append mode, writes the header “Temperature Data Logging” followed by a newline character to start logging data on a new line. The file is automatically closed after writing.
with open('savedata.txt', 'a') as f:
f.write('Temperature Data Logging')
f.write('\n')
Code language: Python (python)
The calcTemp()
function reads the voltage from the internal temperature sensor through the ADC, converts it to Celsius using a specific formula, and stores the result in the global variable temperature_celsius
.
def calcTemp():
global temperature_celcius
ADC_voltage = adc.read_u16() * (3.3 / (65535))
temperature_celcius = 27 - (ADC_voltage - 0.706)/0.001721
Code language: Python (python)
The logData()
function retrieves the current timestamp from the real-time clock (RTC) and checks the size of the “savedata.txt” file. If the file size is less than 20,000 bytes (20KB), it opens the file in append mode, writes the timestamp and temperature data, separated by commas, on a new line, and then closes the file.
If any error occurs during this process, it prints a message indicating that the data could be saved.
def logData():
timeTuple=rtc.datetime()
file_size=os.stat('/savedata.txt')
if(file_size[6]<20000):
try:
with open('savedata.txt', 'a') as f:
f.write('Timestamp='+ str(timeTuple[4])+':'+str(timeTuple[5])+',')
f.write('Temperature='+ str(temperature_celcius))
f.write('\n')
except:
print("Error! Could not save")
Code language: Python (python)
We then call the functions calcTemp()
to read the temperature and also call logData()
to save the temperature data on the savedata.txt file.
calcTemp()
logData()
Code language: Python (python)
The code runs a while
loop where it measures the temperature using the calcTemp()
function, logs the data using logData()
, and updates the timestamp (timeStamp
) every 2 minutes. This loop continuously records temperature data at regular intervals.
while True:
if (time.ticks_ms()-timeStamp)>120000:
calcTemp()
logData()
timeStamp=time.ticks_ms()
Code language: Python (python)
Conclusion
The internal temperature sensor of Raspberry Pi Pico is not so accurate. We used it as an example, but you can use more reliable sensors such as DHT11, DHT22, BME280, and BMP280 for logging data such as temperature, pressure, and humidity.
You can follow our guides below to learn more about interfacing such sensors:
- Raspberry Pi Pico With DHT22 & MicroPython.
- Use DHT11 with Raspberry Pi Pico.
- Use BME280 With Raspberry Pi Pico W & MicroPython Code
- Raspberry Pi Pico with BMP280 – MicroPython Guide
In this guide, we demonstrated how we can log data to the flash memory of Raspberry Pi Pico W. MicroPython code was used to save a text file with temperature data. Hope you found the article helpful. Please leave your thoughts and queries in the comments below.
Leave a Reply