This tutorial will help you to run MicroPython on ESP32 using Thonny IDE. Learn how to flash MicroPython UF2 file, and upload MicroPython code to the ESP32 development board. The steps here are explained using the ESP-WROOM-32 development board and Windows OS. At the end of this tutorial, an example explains how to blink an LED using MicroPython script.
ⓘ If you want to use the uPyCraft IDE instead of Thonny IDE, follow our in-depth guide: Getting Started with MicroPython On ESP32 Using uPyCraft IDE.
Brief Overview Of MicroPython
MicroPython is a port of Python 3 that is specifically designed to work with low-power microcontrollers. It features a subset of the standard Python library and is optimized for bare metal processors. Although MicroPython is not a full implementation of Python, its syntax and features share many similarities.
Once we upload a MicroPython script to a microcontroller, the MicroPython runtime environment, which is a tiny software that runs on the microcontroller’s CPU, interprets the code. The code will be then executed by the MicroPython runtime environment, which also controls memory allocation and grants access to the hardware capabilities of the microcontroller. On the other hand, code like C/C++ is not interpreted but compiled.
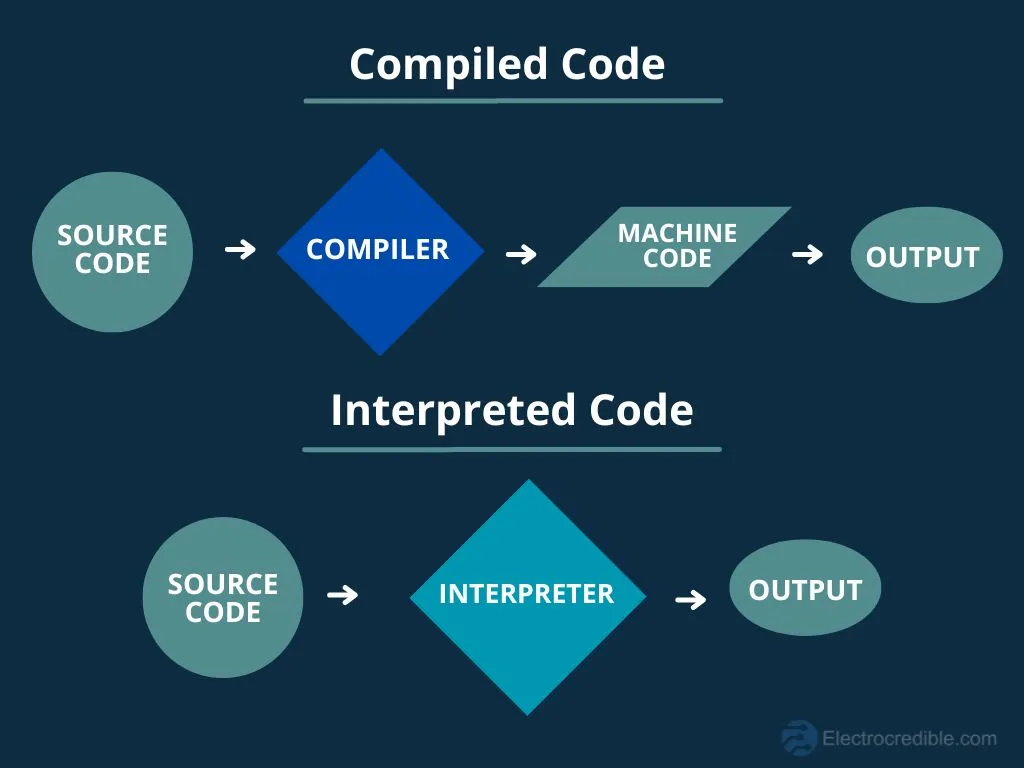
MicroPython has a built-in filesystem and allows us to execute commands via a USB port. We get an interactive prompt called the REPL to interact with the microcontroller. More on the REPL is discussed later in this guide.
Also read: Arduino vs MicroPython vs CircuitPython: Which One Will You Choose?
Many manufacturers release development boards that can run MicroPython. Since running MicroPython is resource-intensive, it is not used with older boards such as the Arduino UNO. Raspberry Pi Pico W and ESP32 are well suited to run MicroPython. You can look at our article that discusses the best MicroPython boards you can use.
Requirements
- Thonny IDE- To flash MicroPython uf2 file and edit and upload code. Its installation and usage are explained here.
- ESP32 development board: I shall be using an ESP-WROOM-32 throughout this tutorial. You can choose any other variant you have.
- A USB cable and a computer running Windows OS.
Installation of Thonny IDE
Go to thonny.org. Download the version suitable for your OS from the website. I installed version 4.1.3 for Windows. This version of Thonny IDE has Python 3.10.11 built-in. So, unlike the installation of uPyCraft IDE, we do not need a separate installation of Python.
Open the installation file once the download completes. Click on Next.
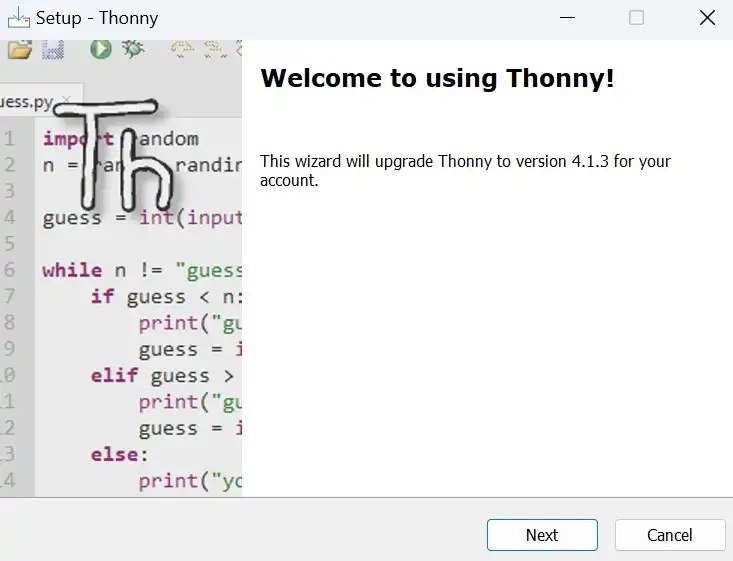
Accept the license agreement and click on Next.
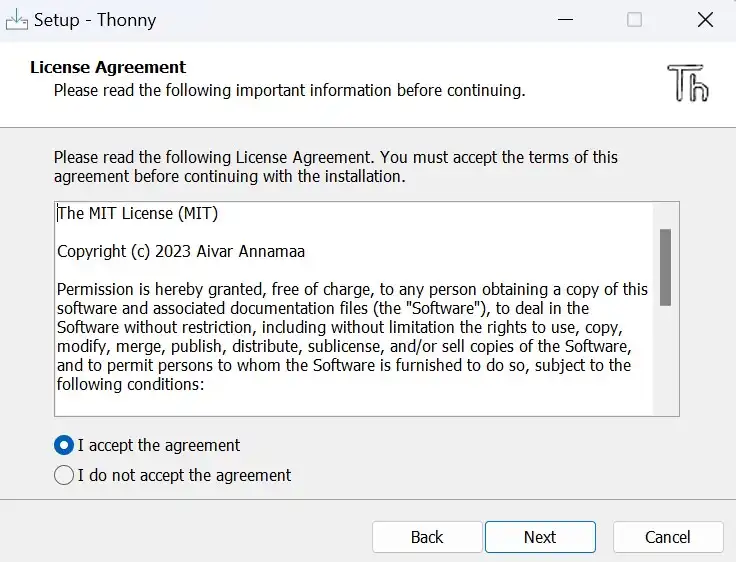
Set a desktop shortcut if you want and also set the location of the Thonny installation. Finally, click on the Install button.
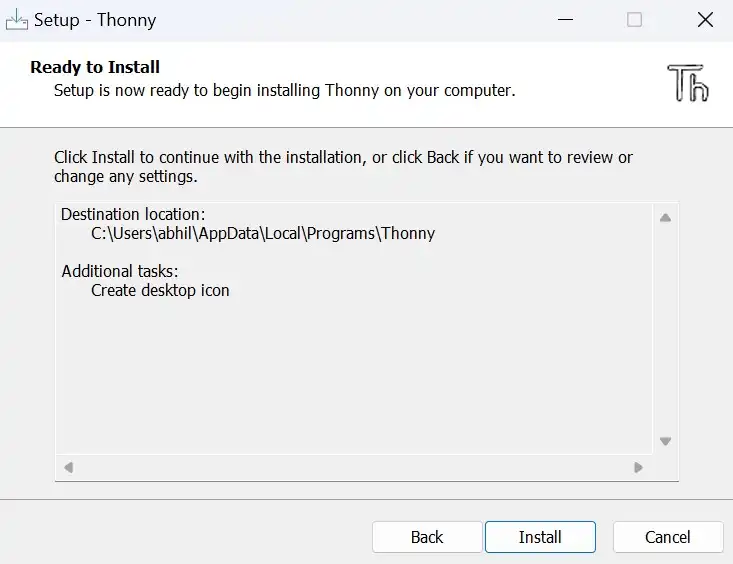
Click on Finish in the last step to complete the installation.
Upon launching the IDE for the first time, set the Initial settings section to ‘Standard‘ and click the ‘Let’s Go‘ button.
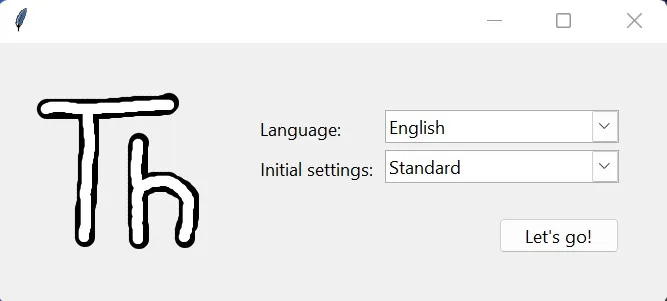
Flashing MicroPython on ESP32 Using Thonny IDE
To run MicroPython code on ESP32, we need to upload a special file with ‘.bin‘ extension to ESP32. This file will be responsible for compiling the MicroPython code to bytecode and features the runtime interpreter to run the bytecode.
Donwload .bin File
Visit the link https://micropython.org/download/ESP32_GENERIC/ and download one of the latest builds for your board. The screenshot below shows the .bin file I downloaded for my ESP-WROOM-32 board.
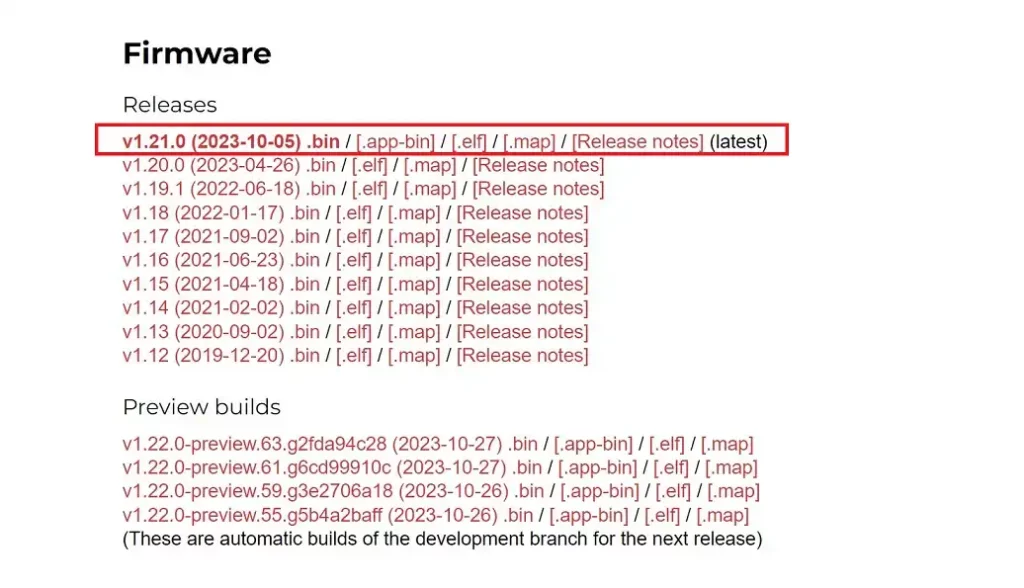
Flash .bin File
Now, the downloaded .bin file has to be uploaded to ESP32 before we can run MicroPython code on it. Follow these steps:
- Connect your ESP32 to your computer using a USB cable.
- In Thonny IDE, go to Tools>Options.
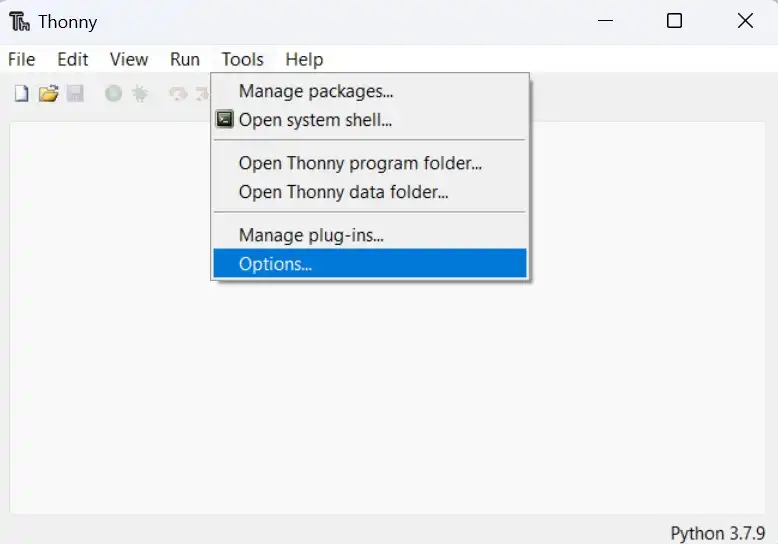
- Select the Interpreter tab. Select ESP32 as the interpreter from the drop-down list. Then click on ‘Install or update MicroPython‘. (If the Port is not visible, refer to the troubleshooting section later in this article).
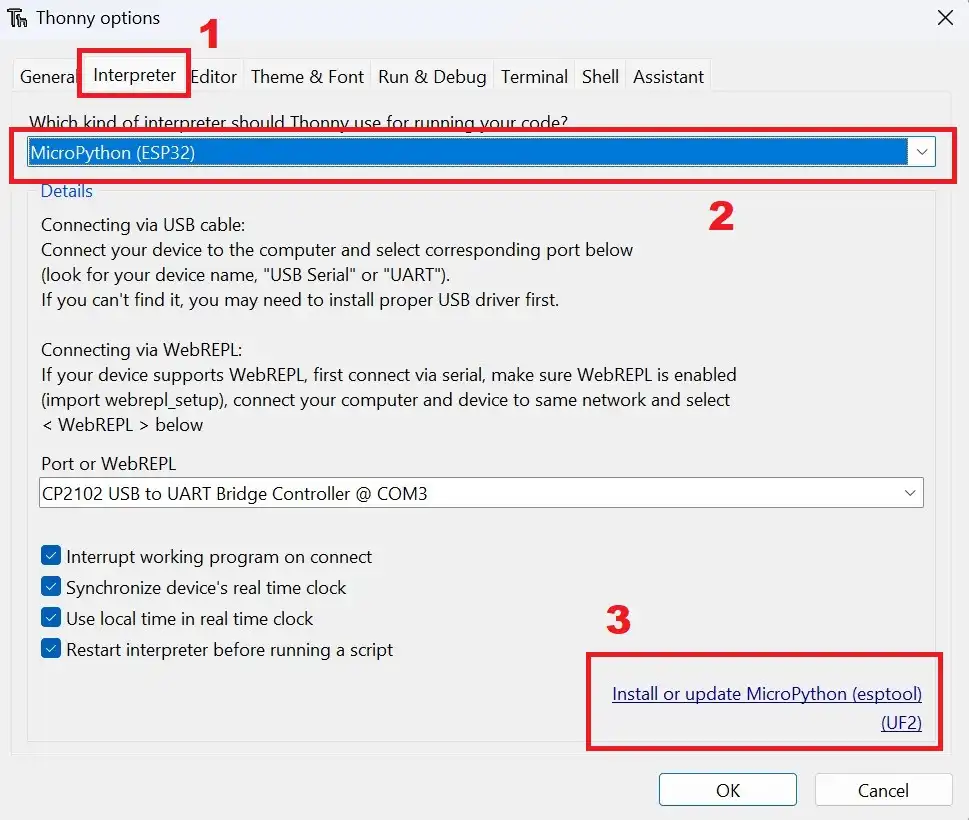
- Wait while “downloading variants info” is shown.
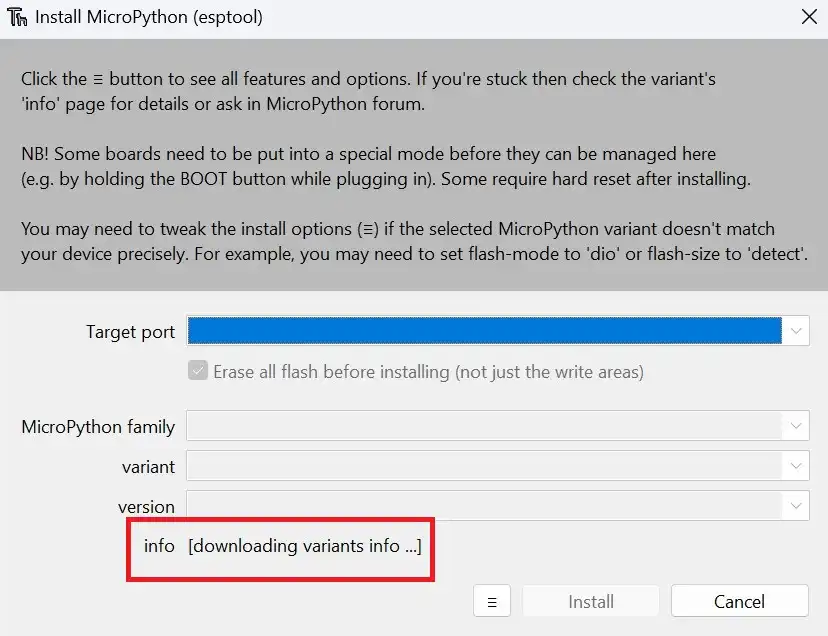
If the “Could not download variants info from” error is shown, you might need to use a VPN for the variants info to be downloaded.
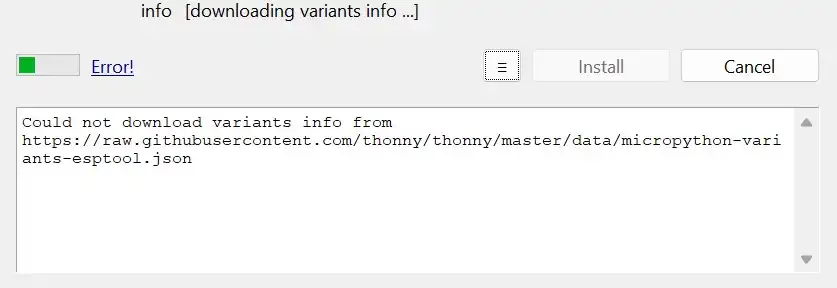
- The ‘Target port‘ section will automatically detect and select your ESP32 board driver. If not, refer to the troubleshooting section below.
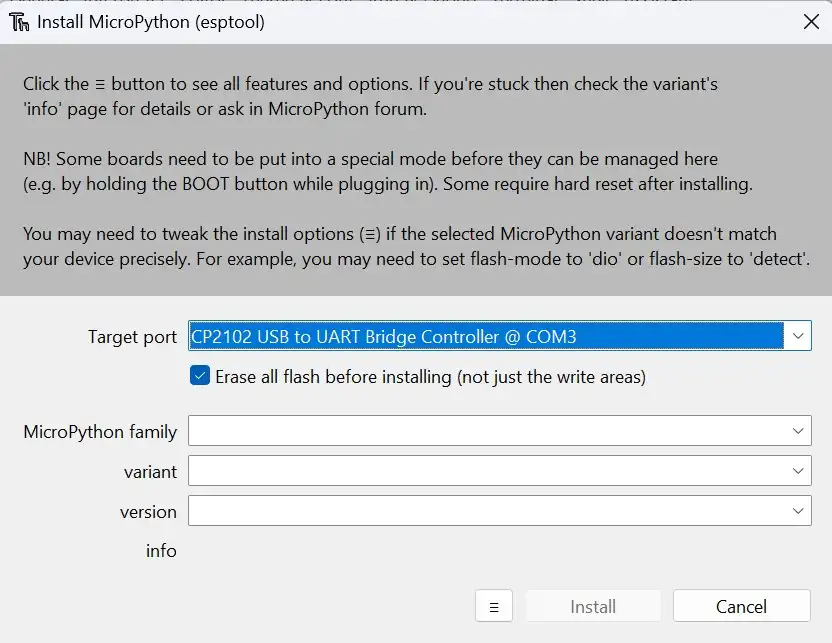
- Click on the ≡ button and then click on “Select local MicroPython image“
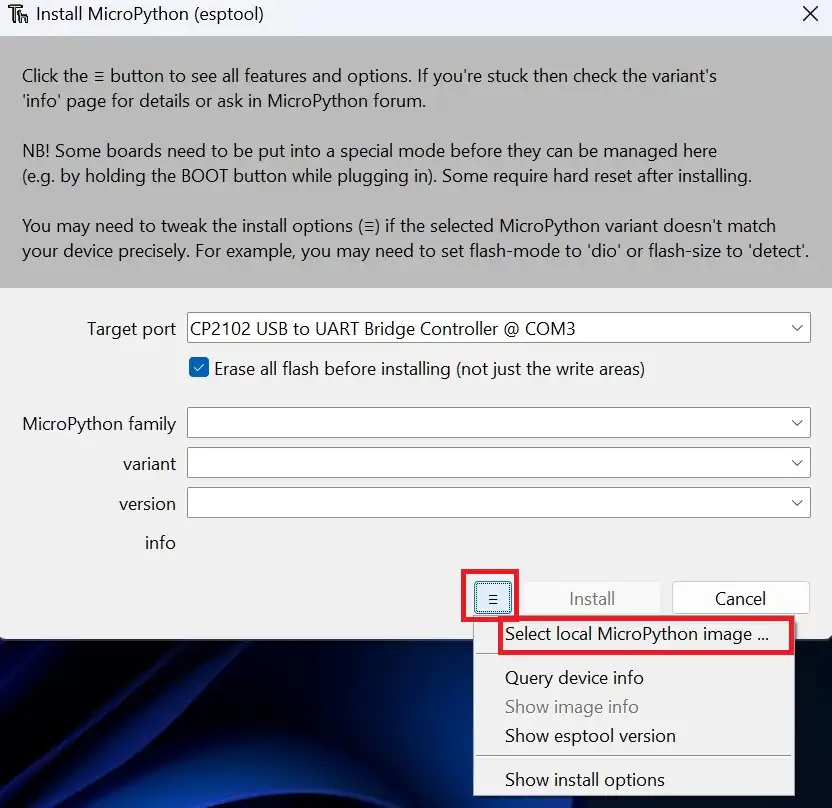
- Browse and select the .bin file downloaded earlier. Click on the install button after the file is loaded.
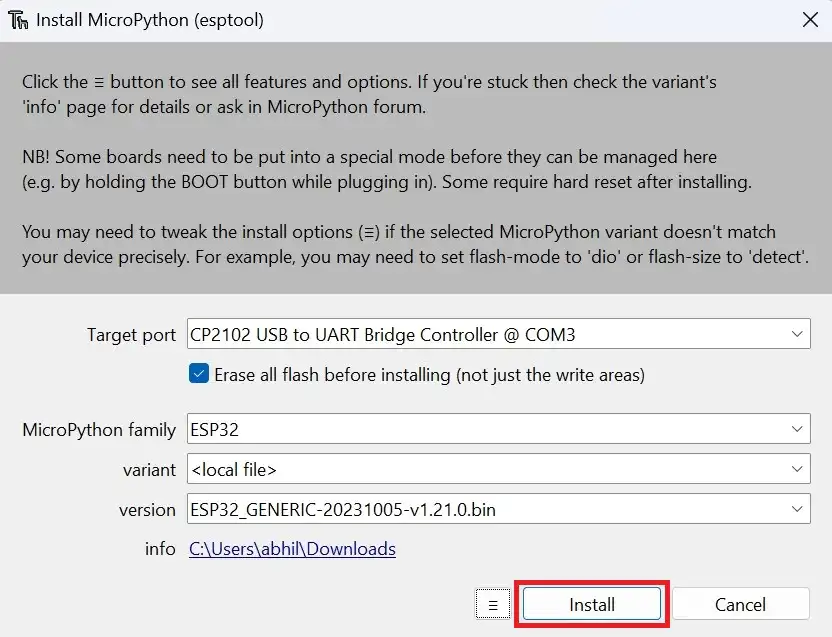
- Wait for some time while the installation completes. A ‘done’ message will be displayed and then you can close the window.
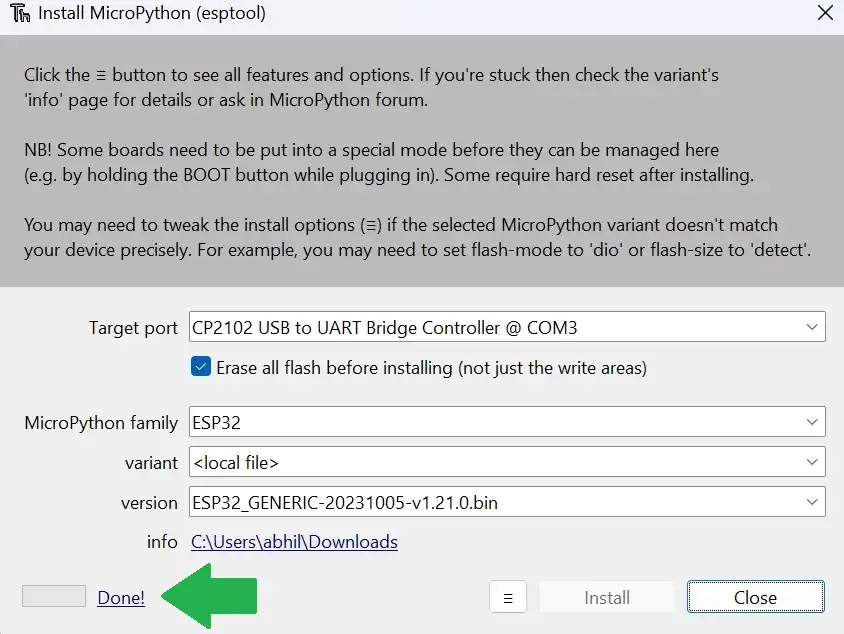
- Restart Thonny IDE when done. Keep your ESP32 connected via USB.
If the installation is successful, the shell in Thonny will show an output as shown below. The active interpreter in Thonny will also be shown in the bottom right corner of the IDE.
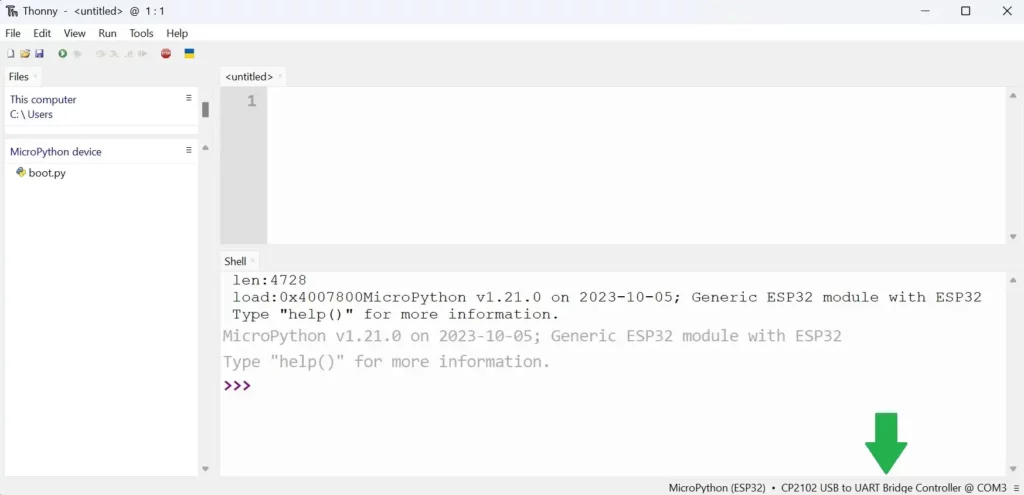
When the >>> symbol is shown in the shell of Thonny, the MicroPython REPL is ready to accept commands.
Testing MicroPython REPL in Thonny IDE
If we power up an ESP32 with MicroPython installed on it, it waits for user commands to perform an action. The fastest way to interact with it is through REPL.
REPL stands for read-evaluate-print loop. In the read phase, MicroPython waits for code to be written on the shell of an IDE. Upon pressing the Enter key, Micropython evaluates the typed code and runs it immediately. If any result is to be shown, it is printed for the user to view. This process continues in a loop, i.e. it again goes back to the read phase and waits for the next line of code to be written.
Type print('hello')
in the Thonny shell, next to the >>> symbol.
The shell will print hello in the next line.
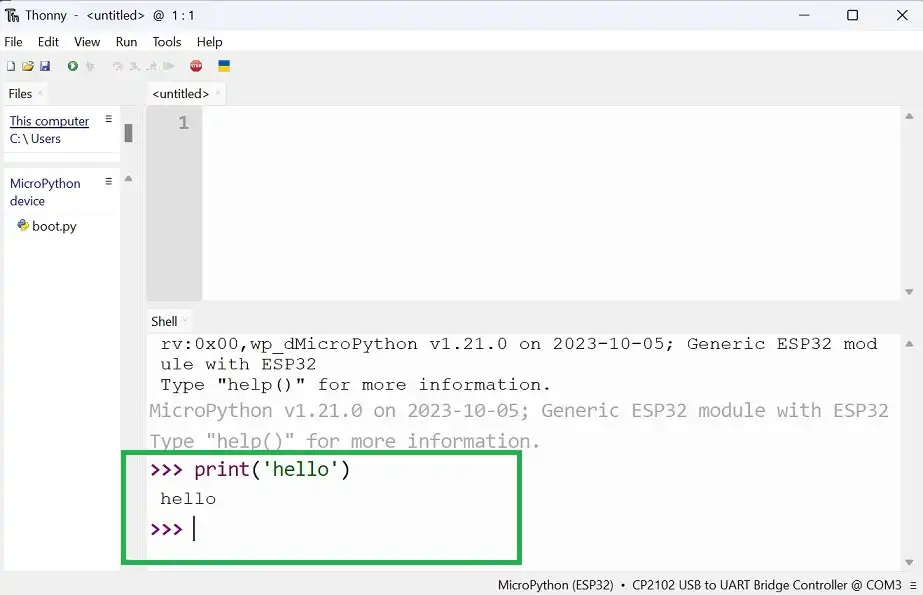
This action will verify that MicroPython runtime is successfully installed on your ESP32.
MicroPython Code Upload Using Thonny IDE
Now that we have verified the MicroPython REPL works onboard the ESP32, let us write a simple MicroPython script and upload it to ESP32. The script will blink the LED onboard the ESP32 development board.
First, ensure that Thonny IDE is set to use the MicroPython interpreter on ESP32. Go to Tools>Options>Interpreter in Thonny IDE, and set the interpreter to ESP32. Also ensure the correct COM port is selected.
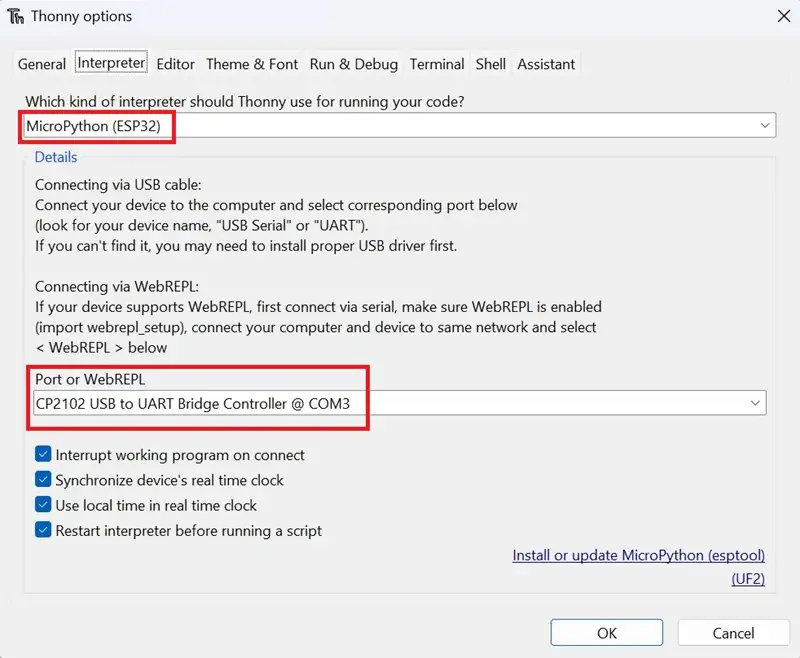
ⓘ You may need to set the interpreter again the next time you open Thonny IDE.
Create a new file in Thonny IDE. You can use the shortcut ‘Ctrl+N’ or go to File>New.
Find the pin number for the onboard LED on the ESP32 board you are using. ESP-WROOM-32 board that I used has pin 2 connected to the onboard LED. Copy and paste the following code in the code editor space. You might need to change the line led_pin=2
depending on your board.
from machine import Pin
import time
led_pin=2
led = Pin(led_pin, Pin.OUT)
while True:
led.value(0)
time.sleep_ms(500)
led.value(1)
time.sleep_ms(500)
Code language: JavaScript (javascript)
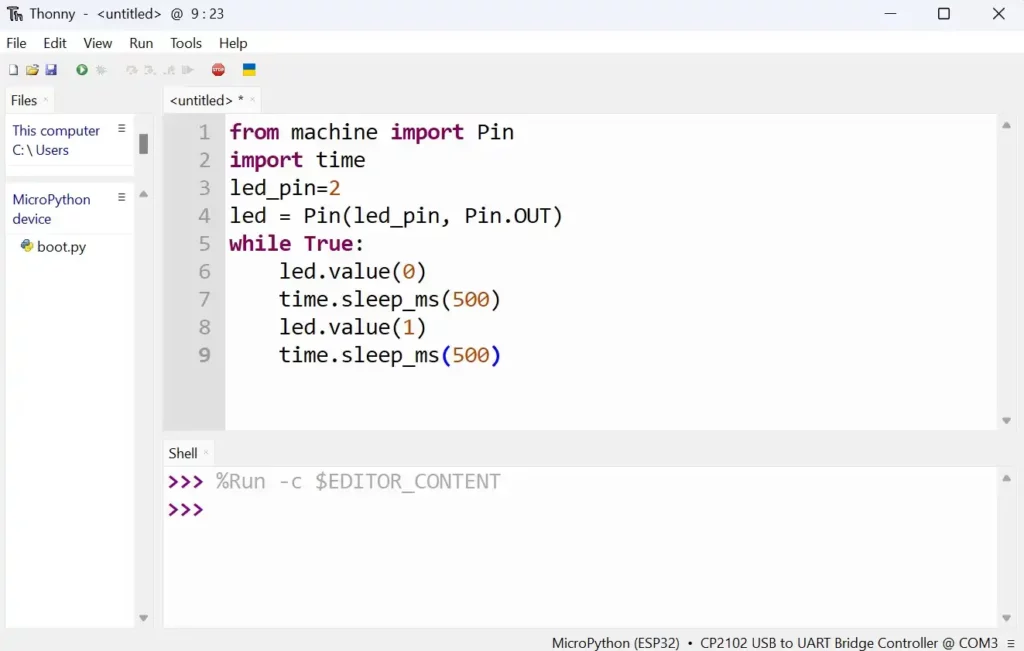
Click on File>Save as from the top toolbar in Thonny.
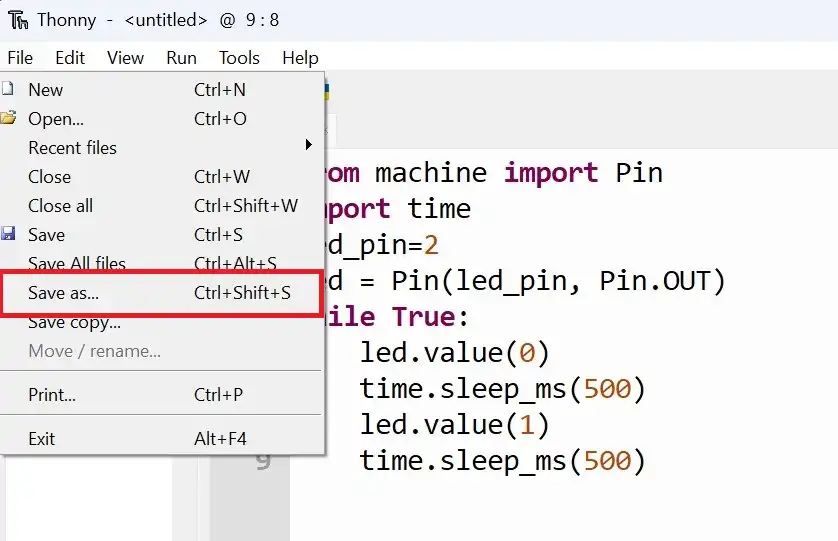
On the pop-up window, select MicroPython device as the save location.
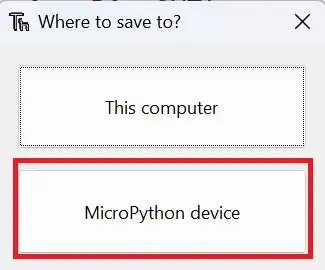
Set the filename as main.py and click OK.
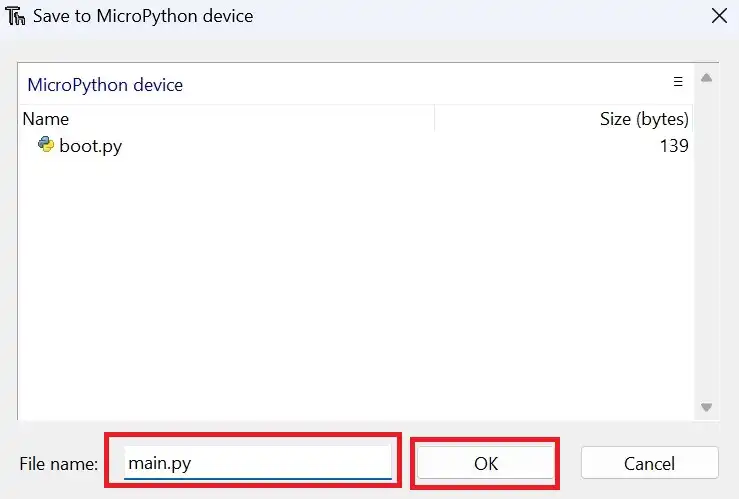
Click on the Run button in the toolbar or press F5.
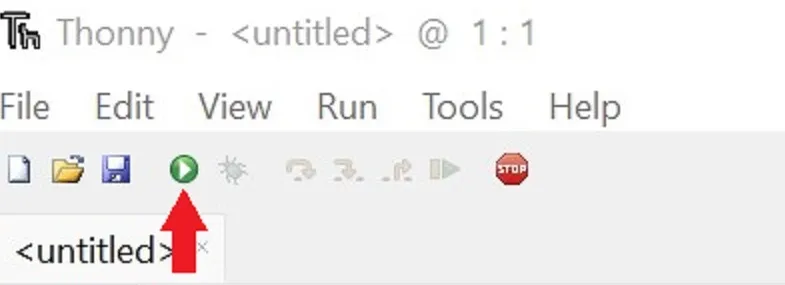
The LED onboard your ESP32 board must now start blinking.
Code Explanation
First, import the necessary MicroPython. Import the Pin
class from the machine
module, which is used for hardware control. Import the time
module for time-related functions.
from machine import Pin
import time
Code language: JavaScript (javascript)
Define the pin number (led_pin
) where the LED is connected. In this case, it’s set to pin 2, as the ESP-WROOM-32 board has GPIO 2 connected to the onboard LED. Your ESP32 board may have a different pin connected to the LED. Look for the pinout of your ESP32 model using a web search and change this line if required.
led_pin=2
Create a Pin
object named led
and configure it as an output pin using Pin.OUT
. This object will be used to control the LED.
led = Pin(led_pin, Pin.OUT)
Enter an infinite loop using while True:
. This ensures that the following code will run continuously until manually stopped or interrupted.
while True:
Code language: PHP (php)
Inside the loop, the LED is turned OFF by setting the pin’s value to 0.
led.value(0)
Code language: CSS (css)
A delay of 500 milliseconds is introduced using. This keeps the LED in the OFF state for 0.5 seconds.
time.sleep_ms(500)
Code language: CSS (css)
The LED is then turned ON by setting the pin’s value to 1. Another delay of 500 milliseconds is introduced, keeping the LED in the ON state for 0.5 seconds.
led.value(1)
time.sleep_ms(500)
Code language: CSS (css)
The code essentially creates a simple LED blinker that alternates between turning the LED on and off with a 1-second (500 ms on, 500 ms off) cycle. This process repeats indefinitely within the while True:
loop, creating a continuous blinking effect.
Troubleshooting
If the COM port is not visible in the Thonny IDE then the proper driver for the USB communication chip onboard ESP32 module might be missing. To verify that the drivers are properly installed:
- After connecting your ESP32 to the computer via a USB cable, go to Device Manager and expand the PORTS section. As I have the proper drivers, Silicon Labs CP210x USB to UART Bridge (COM3) is being shown.
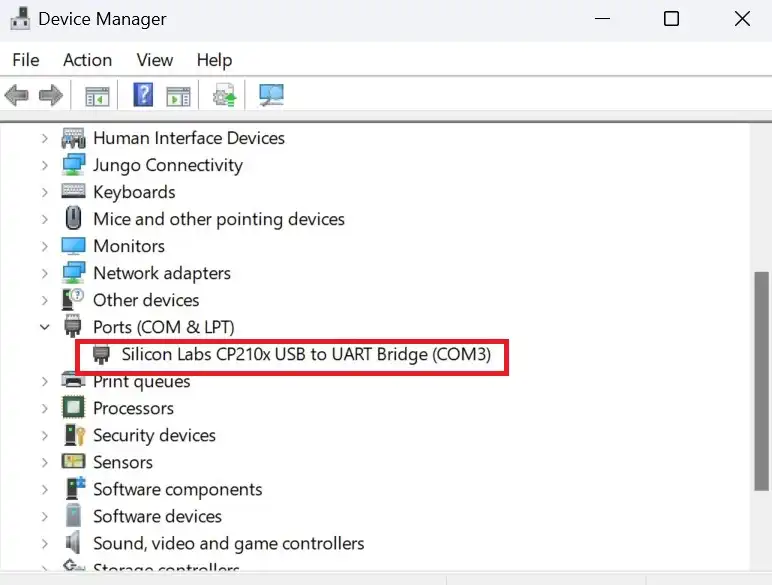
- If no such device is visible under the PORTS section, then you will have to manually install the correct drivers.
- First, find out the chip responsible for USB communication on your development board. It is often located near the USB port.
- In the ESP-WROOM-32 board I used, the Silicon Labs CP2102 chip is used as shown in the photo below. Some ESP32 boards also use the CH340 chip.
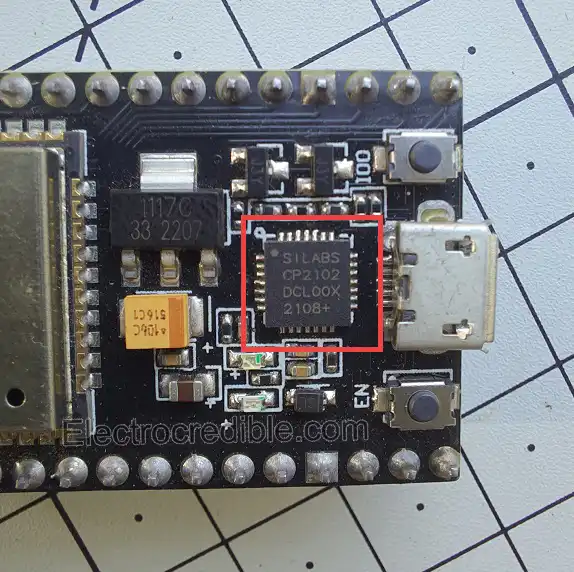
- Find the driver required by searching the web for “chip name + driver”. Alternatively, you can follow this guide to install CH340 chip drivers or visit the Silabs website to download the driver for the CP210X chip.
- After installing the correct driver, go to Device Manager and verify that the driver is visible under the PORTS section. Now upon restarting your IDE, the COM port must be visible.
- If this method fails, ensure the USB cable is working properly. Some USB cables are only meant for charging devices while others can carry data too. You will need a USB data cable for communicating with ESP32.
Conclusion
In this guide, we discussed how we can easily flash MicroPython on ESP32 using Thonny IDE. We also tried an example of running a main.py MicroPython file. You can take a look at our other MicroPython articles for more experimentation.
Also read: Getting Started: MicroPython On ESP32 Using uPyCraft IDE
Leave a Reply