This article compares the Arduino, MicroPython & CircuitPython programming languages which are used extensively in open-source electronics development. These languages have their strengths and weaknesses which are explained here in layman’s terms. By reading this post, you shall be able to make an informed decision on which language to use for your next project.
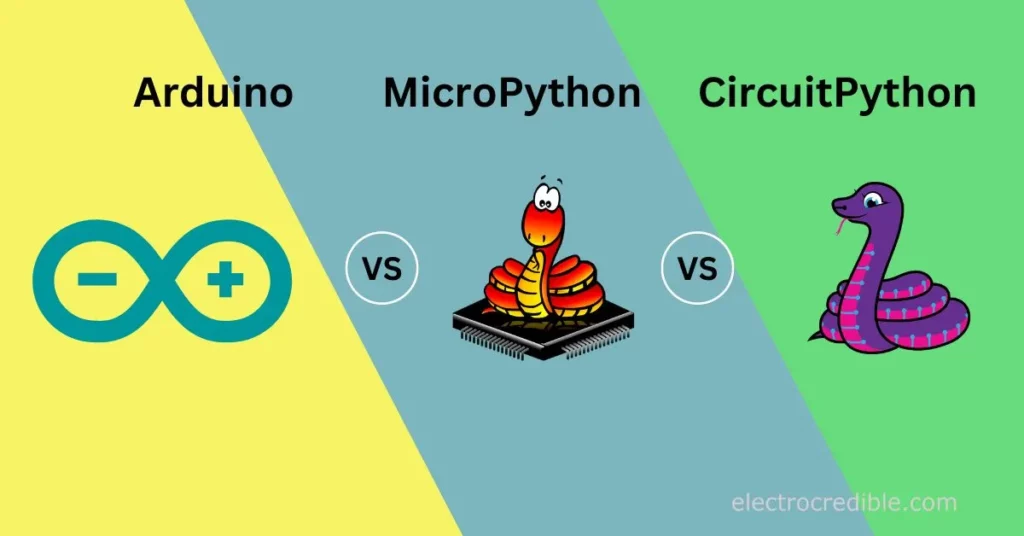
Introduction to Arduino, MicroPython, and CircuitPython
The word ‘Arduino‘ may refer to an Arduino development board(e.g. Arduino UNO), the Arduino programming language based on C/C++, or the Arduino Development Environment(IDE) which is used to write and upload sketches to a development board. Together, they form the open-source Arduino ecosystem that helps beginners to get started with microcontroller programming. The ease and simplicity of the Arduino platform have made it popular among hobbyists and professionals alike.
MicroPython is an implementation of the Python programming language, optimized to run on microcontrollers. After a successful Kickstarter campaign in 2013, Australian programmer and theoretical physicist Damien George developed MicroPython. The two components of MicroPython are a compiler for Python to bytecode and a runtime interpreter for the bytecode. Its various modules help to interact with the underlying hardware of a microcontroller. Raspberry Pi Pico and ESP32 are two popular development boards that can be programmed using MicroPython.
CircuitPython is another derivative of the Python programming language created specifically for microcontrollers. It is a fork of MicroPython. Adafruit Industries created it in 2017 and makers have since embraced it. Similar to MicroPython, CircuitPython comprises a Python compiler for bytecode and an on-chip microcontroller runtime interpreter for that bytecode. Its open-source development community helps users to access a variety of libraries and examples for dealing with hardware components.
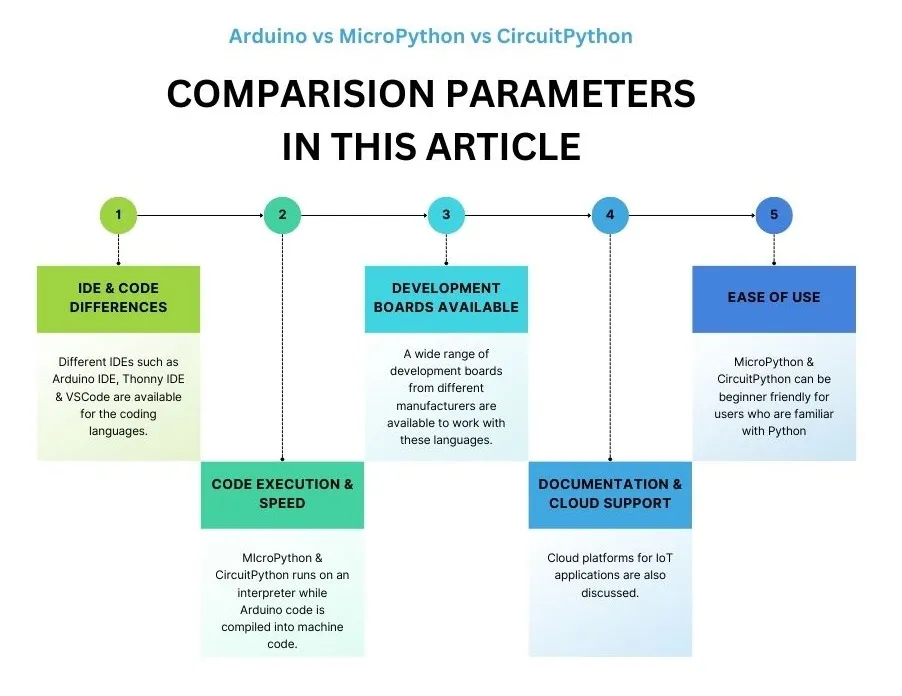
IDE Support
The most popular IDE to program Arduino development boards is the Arduino IDE. While the earlier version lacked many features, the newer version of the IDE supports many advanced features such as code autocompletion, syncing with Arduino Cloud, and a built-in debugger.
Another popular alternative is to program Arduino boards using the PlatformIO IDE, which is built upon VSCode. Some other popular alternatives to the Arduino IDE are:
- Atmel Studio, an IDE specifically designed for programming Atmel microcontrollers.
- CodeBlocks, an open-source, cross-platform IDE.
- Eclipse IDE has a plugin called Sloeber that provides support for programming Arduino boards.
Using Arduino Web Editor, we can write, compile, and upload code to any official Arduino board right from a web browser.
For MicroPython, Thonny IDE and uPyCraft IDE are two of the most popular ones. If you are comfortable with using VSCode, there is an external plugin called Pymakr that brings MicroPython support to VSCode. Some alternative IDEs to program in MicroPython are:
- Pycharm.
- microIDE.
Unlike Arduino and MicroPython, we do not an IDE in the case of CircuitPython. Boards with CircuitPython installed show up in the computer as a USB drive called CIRCUITPY. A code.py file which is located on the CIRCUITPY drive is where we need to save our code. A code editor can be used to write and edit CircuitPython code and save it in the code.py file.
Mu Editor is one of the recommended editors to write CircuitPython code for Adafruit boards. Some other alternative editors are:
- GNU Emacs.
- VSCode.
- Sublime Text.
Code Differences: Arduino vs MicroPython vs CircuitPython
In this section, we will see how the code to blink an LED differs in the 3 languages.
Arduino Code
Below is the code to blink the onboard LED in a Raspberry Pi Pico board using code written in Arduino IDE:
void setup() {
pinMode(LED_BUILTIN, OUTPUT); // initialize digital pin LED_BUILTIN as an output.
}
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}
Code language: C++ (cpp)
The code inside setup()
function will execute only once at startup. Thereafter, the code inside loop()
function will continue to execute indefinitely. The loop()
function resembles while(1) in C++.
MicroPython Code
Here is the MicroPython code to blink the onboard LED in Raspberry Pi Pico.
from machine import Pin
import time
#initialize pin connected to onboard LED as output.
led = Pin("LED", Pin.OUT)
while True:
led.low()
time.sleep(1)
led.high()
time.sleep(1)
Code language: Python (python)
In MicroPython, code is interpreted line by line by the MicroPython interpreter in runtime. Notice that in Arduino IDE, we did not have to include any libraries to blink an LED. This is because the Arduino IDE takes care of necessary libraries required for I/O and compiles them even if they are not explicitly called in our program.
CircuitPython Code
Below is the CircuitPython code to blink an LED continuously. Its syntax has some differences from MicroPython.
import time
import board
import digitalio
#initialize pin connected to onboard LED as output.
led = digitalio.DigitalInOut(board.LED)
led.direction = digitalio.Direction.OUTPUT
while True:
led.value = 1
time.sleep(1)
led.value = 0
time.sleep(1)
Code language: Python (python)
Also read: Raspberry Pi Pico vs Arduino – Which Board To Choose?
Code Execution
Code written in Arduino IDE is compiled into machine code that can run on the microcontroller embedded in the Arduino board. This generated code can be regarded as “native” to the hardware platform because it was created specifically for the microcontroller’s hardware architecture.
Once we upload a MicroPython script to a microcontroller, the MicroPython runtime environment, which is a tiny software that runs on the microcontroller’s CPU, interprets the code. The code will be then executed by the MicroPython runtime environment, which also controls memory allocation and grants access to the hardware capabilities of the microcontroller.
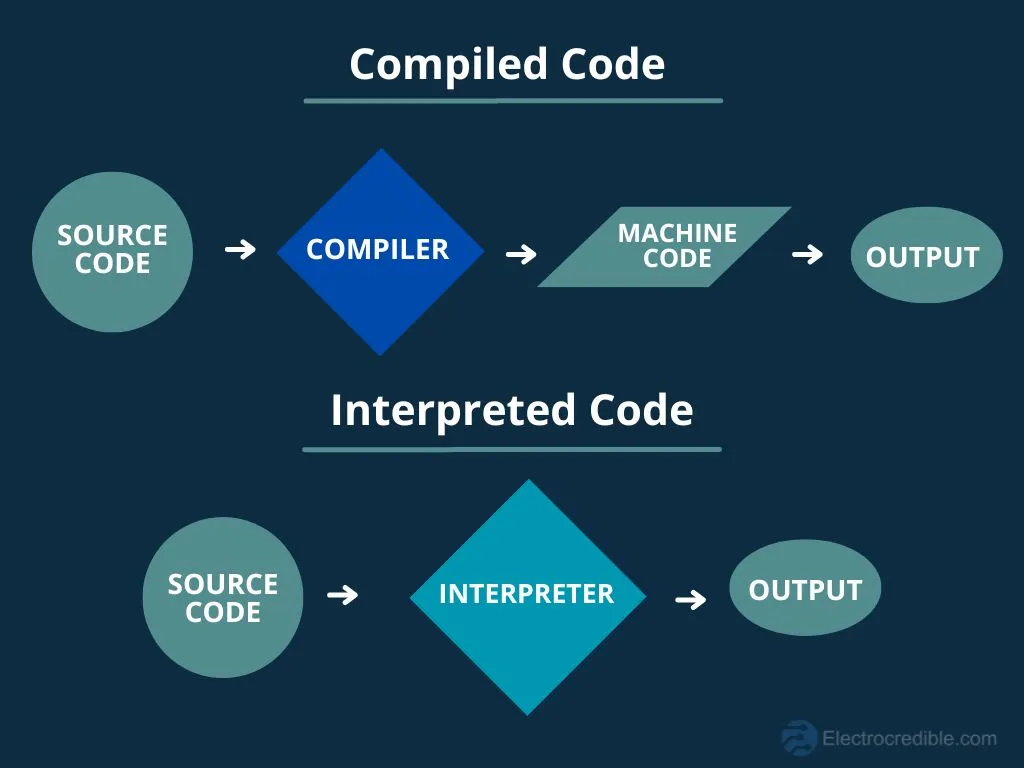
Similarly, in the case of CircuitPython, a program is initially translated into bytecode after being written. CircuitPython runtime environment takes care of code execution and memory allocation/deallocation.
Which Is Faster? Speed Comparision
As Arduino code is compiled into machine code that is tailored for the microcontroller’s architecture, it is generally faster than MicroPython code which is interpreted at runtime. C/C++, a compiled language, is typically used to create Arduino code. Since C/C++ code can be highly optimized, its execution is fast and efficient. Despite the fact that MicroPython offers a number of performance-enhancing features, interpreted code runs slower than compiled code.
However, for simple projects such as reading sensor data or home automation, differences in code execution speed should not matter. But for advanced projects such as audio projects, code execution speed should be taken into concern.
Also read: Getting Started With Raspberry Pi Pico & Raspberry Pi Pico W Using MicroPython
Development Boards: CircuitPython vs MicroPython vs Arduino
The Arduino IDE ecosystem supports an extensive range of development boards from different manufacturers. Among them, some of the boards manufactured by Arduino are Arduino Uno, Arduino Nano, Arduino Mega, Arduino Due, Arduino Leonardo, Arduino Pro Mini, Arduino Micro, Arduino Mini, Arduino Zero, Arduino MKR series, Arduino Nano Every, and Arduino Portenta H7.
Boards featuring the ESP32 and ESP8266 MCUs can also be programmed using Arduino IDE. Some of them are ESP32 Dev Module, ESP32 Thing Development Board, SparkFun ESP32 Thing, ESP32 Wrover Module, ESP32 Pico Kit, NodeMCU-32S, and TTGO T8 ESP32-S2.
The RP2040 microcontroller boards Raspberry Pi Pico and Raspberry Pi Pico W can be programmed using Arduino IDE. Moreover, RP2040 boards from different manufacturers such as Adafruit, Invector Labs, Sparkfun, and Waveshare are also supported.
A development board called the pyboard is the official board to run MicroPython. It features an STM32F405RG microcontroller. Raspberry Pi Pico, ESP32, and ESP8266 are among the most popular boards that run MicroPython.
CircuitPython is also supported in a wide variety of boards, most of which are developed by Adafruit. Raspberry Pi Pico, Arduino Nano RP2040 connect, and Seeed Studio XIAO RP2040 are some other boards supporting MicroPython. You can visit the downloads sections of CircuitPython to view all the boards that can run CircuitPython.
Cloud Support
Arduino has cloud capabilities through the Arduino Create platform. Arduino Cloud enables you to remotely program and monitor your Arduino boards. It becomes easier to collaborate with others using the Arduino Cloud. When writing this article, the free version of Arduino Cloud supports up to two devices.
CircuitPython Adafruit IO is a module that can help to communicate with Adafruit IO using either the HTTP API or the MQTT API. Adafruit IO is a cloud service using which you can store and retrieve data, read sensors, and control devices via the internet. It has a free plan in addition to a paid plan.
Documentation & Community Support
There is a large community of Arduino users and many groups/pages/subreddits in social media where users share and discuss projects. As MicroPython and CircuitPython are relatively new, their community is not as large as Arduino, but steadily growing. You should not have a problem troubleshooting most issues as you would often find answers with a Google search. MicroPython has a bigger community as it has been around longer than CircuitPython.
All the languages are well documented on their respective official websites.
Ease Of Use – MicroPython vs CircuitPython vs Arduino
One of the primary advantages of using MicroPython/CircuitPython is that you can write code in a high-level, simple language like Python while having access to low-level hardware capabilities. This makes it perfect for quick and easy project development and experimentation, as well as for creating programs that can run with the limited resources of microcontrollers.
CircuitPython offers some benefits over MicroPython, such as a standardized module/library collection and a simplified syntax that make it more accessible to beginners. Programming using CircuitPython is also easier with just a text editor. Moreover, we can drag/drop files with ease as the files show up on a USB drive if CircuitPython is installed on your board.
By looking at the code, a learner with little experience should be able to comprehend how a MicroPython/CircuitPython program works. Python has risen in popularity due to its ease of use, and beginners find it easier to understand.
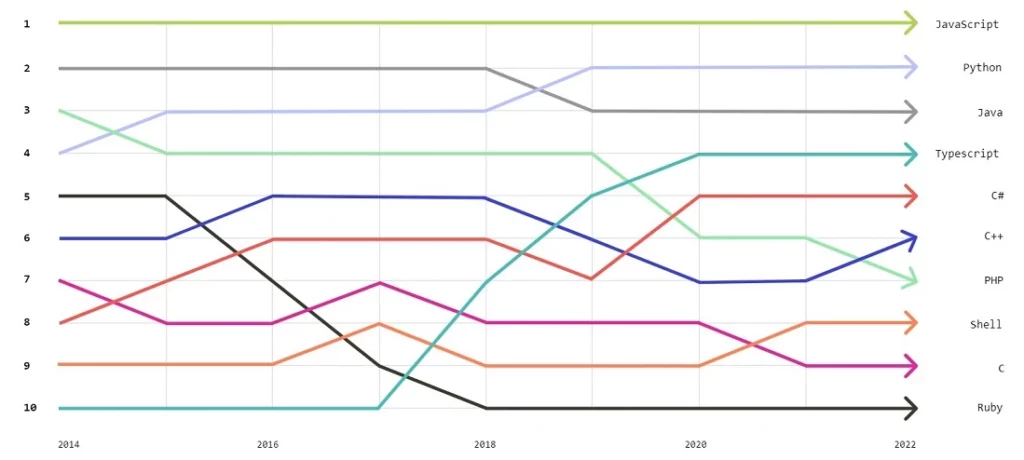
Python was the second most popular language in 2022. However, if you are unfamiliar with the Python programming language and have prior experience with C/C++/Java, getting started with the Arduino platform will be easier for you. There are a lot of libraries available for Arduino that can get you quickly started with your projects.
Which One To Use? Which is Better?
C/C++, upon which Arduino code is based, is faster than MicroPython, but more difficult to learn. MicroPython/CircuitPython is easier to understand, but it is slower. All three languages have their advantages and disadvantages, with MicroPython/CircuitPython being flexible due to its syntax and modules, while Arduino code runs faster.
You can use Arduino code if:
- You are familiar with programming languages like C/C++/Java.
- The code is time-sensitive and the speed of the program matters. For example, projects involving FFT, audio processing, real-time data processing, fast ADC/DAC, etc.
- The development board you are using has limited memory.
Note that Arduino IDE also adds some overhead to your code. If speed is of concern, then it is best to avoid Arduino and code in bare metal using ‘Embedded C’ or Assembly.
You can use MicroPython/CircuitPython if:
- You are a beginner in the field of embedded electronics and have no significant experience in coding microcontrollers.
- You are familiar with the Python programming language.
Which one of these languages should you use? The answer depends on the unique project requirements, existing libraries and modules, how fast your program has to run, the programming languages you are comfortable with, and the documentation and community support of the languages. I hope that by reading this article on CircuitPython vs MicroPython vs Arduino, you will be able to select which platform to use in your next project.
Thank you for reading. Leave your thoughts in the comments below.
Read Next:
Leave a Reply